In the previous example, we demonstrated how to take the RG device and output it to the computer.
It would be better if we, instead of having one string, parse the data sent by our device.
Using the previous code let’s add to it and convert the data into numbers.
Principle
The incoming serial data looks something like this:
Acc 0.000 in, EventAcc 0.004 in, TotalAcc 0.363 in, RInt 0.000 iph, XTBTips 0, XTBEventAcc 0.00 in, XTBTotalAcc 0.00 in, XTBInt 0.00 iph
Our goal is to parse these values into specific float-point types.
This is the same Reference Diagram from before.
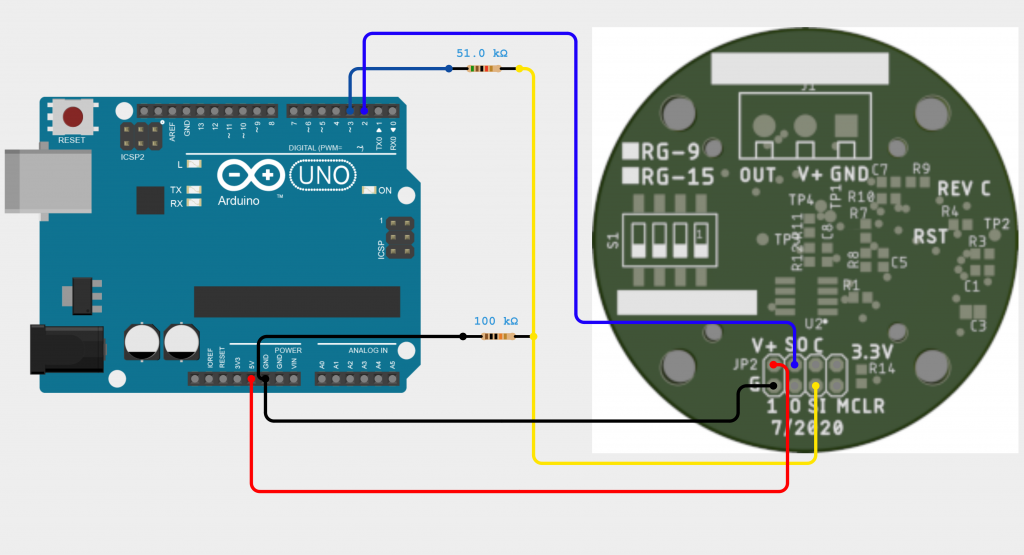
Code
Instead of calling Serial.println(response), let’s take the data and parse it. We need to create some variables to hold our data, all of which are character arrays:
char acc[7], eventAcc[7], totalAcc[7], rInt[7], unit[4];
sscanf (response.c_str(),"%*s %s %[^,] , %*s %s %*s %*s %s %*s %*s %s", &acc, &unit, &eventAcc, &totalAcc, &rInt);
sscanf takes a given string with a format template provided. %s above represents a string and when it is %*s it tells us to read that string but to not use it.
The data stored in the char variables can now be used to print and also be converted to floats. for example to convert acc to a float:
float accFloat = atof (acc);
Printing the entire thing is up to you but this is how the tutorial presents it:
Serial.print("Accumulation: ");
Serial.print(atof (acc),3);
Serial.println(unit);
Serial.print("Event Accumulation: ");
Serial.print(atof (eventAcc),3);
Serial.println(unit);
Serial.print("Total Accumulation: ");
Serial.print(atof (totalAcc),3);
Serial.println(unit);
Serial.print("IPH: ");
Serial.print(atof (rInt), 3);
Serial.println(" IPH\n");
delay(1000);
Full Code
#include <SoftwareSerial.h>
SoftwareSerial unoSerial = SoftwareSerial(2,3);
void setup() {
Serial.begin(9600);
unoSerial.begin(9600);
}
void loop() {
unoSerial.write('r');
unoSerial.write('\n');
String response = unoSerial.readStringUntil('\n');
char acc[7], eventAcc[7], totalAcc[7], rInt[7], unit[4];
sscanf (response.c_str(),"%*s %s %[^,] , %*s %s %*s %*s %s %*s %*s %s", &acc, &unit, &eventAcc, &totalAcc, &rInt);
Serial.print("Accumulation: ");
Serial.print(atof (acc),3);
Serial.println(unit);
Serial.print("Event Accumulation: ");
Serial.print(atof (eventAcc),3);
Serial.println(unit);
Serial.print("Total Accumulation: ");
Serial.print(atof (totalAcc),3);
Serial.println(unit);
Serial.print("IPH: ");
Serial.print(atof (rInt), 3);
Serial.println(" IPH\n");
delay(1000);
}
Once you run the code the terminal should show this:
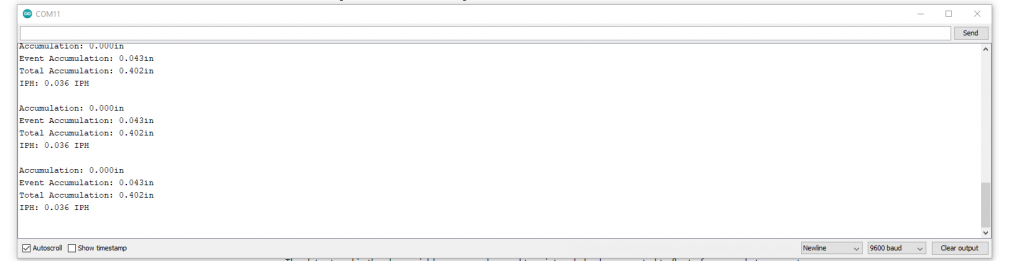