This example assumes you have completed the Basic Serial tutorial.
Polling the device for code is simple but unnecessary for many use cases. When the Rain Gauge is set in continuous mode it will send data every time the accumulation increases. Instead of sending a poll command we just open a stream and frequently read data put into it from the RG.
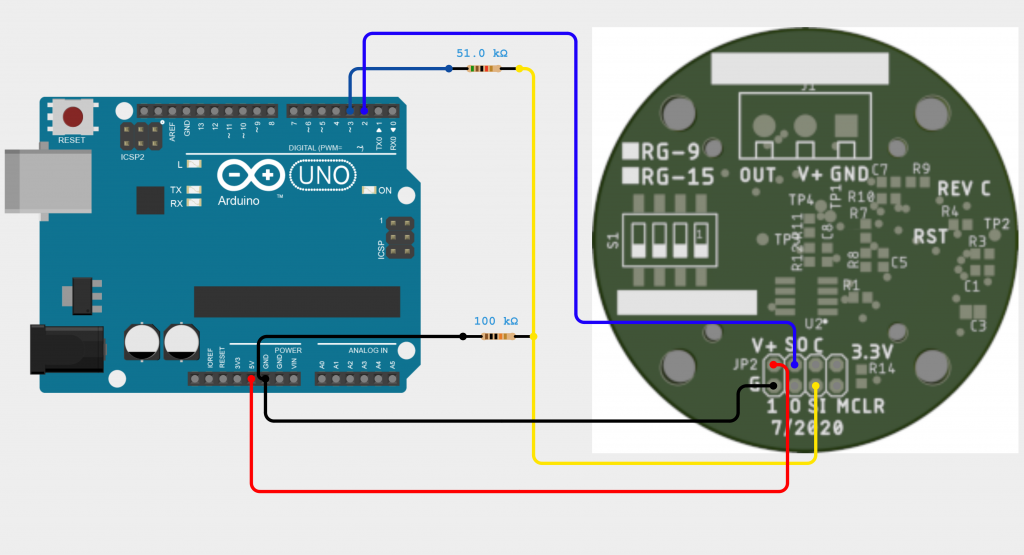
Code
This code is almost identical to the previous example with a few key differences. First thing we want to do is to tell the RG we want to use it in continuous mode with the command ‘c’ and ‘\n’:
void setup() {
...
unoSerial.write('c');
unoSerial.write('\n');
}
In our loop we want to frequently check the stream for any new data in need of processing. If any of the incoming data starts with “Acc” important information must be parsed from the string:
void loop() {
String response = unoSerial.readStringUntil('\n');
if(response.startsWith("Acc")) {
...
}
}
The if statement contains the business logic we want to run with the data. In this example the string is parsed into variables and then printed one at a time:
char acc[7], eventAcc[7], totalAcc[7], rInt[7], unit[4];
sscanf (response.c_str(),"%*s %s %[^,] , %*s %s %*s %*s %s %*s %*s %s", &acc, &unit, &eventAcc, &totalAcc,&rInt);
Serial.print("Accumulation: ");
Serial.print(atof (acc),3);
Serial.println(unit);
Serial.print("Event Accumulation: ");
Serial.print(atof (eventAcc),3);
Serial.println(unit);
Serial.print("Total Accumulation: ");
Serial.print(atof (totalAcc),3);
Serial.println(unit);
Serial.print("IPH: ");
Serial.print(atof (rInt), 3);
Serial.println(" IPH\n");
If all goes well the console should display something like this everytime accumulation is incremented:
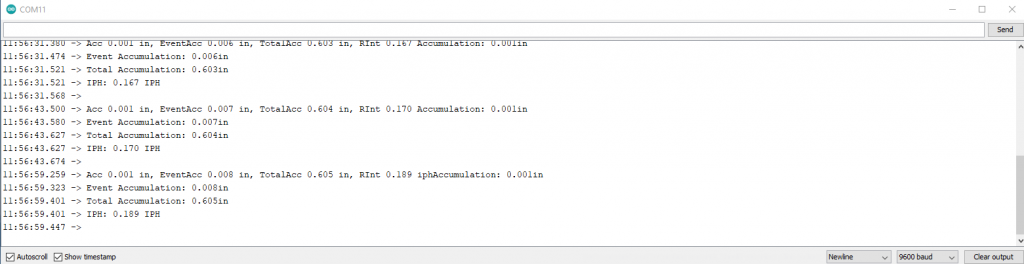
Full Code
#include <SoftwareSerial.h>
SoftwareSerial unoSerial = SoftwareSerial(2,3);
void setup() {
Serial.begin(9600);
unoSerial.begin(9600);
unoSerial.write('c');
unoSerial.write('\n');
}
void loop() {
String response = unoSerial.readStringUntil('\n');
if(response.startsWith("Acc")) {
char acc[7], eventAcc[7], totalAcc[7], rInt[7], unit[4];
sscanf (response.c_str(),"%*s %s %[^,] , %*s %s %*s %*s %s %*s %*s %s", &acc, &unit, &eventAcc, &totalAcc, &rInt);
Serial.print("Accumulation: ");
Serial.print(atof (acc),3);
Serial.println(unit);
Serial.print("Event Accumulation: ");
Serial.print(atof (eventAcc),3);
Serial.println(unit);
Serial.print("Total Accumulation: ");
Serial.print(atof (totalAcc),3);
Serial.println(unit);
Serial.print("IPH: ");
Serial.print(atof (rInt), 3);
Serial.println(" IPH\n");
}
delay(3000);
}